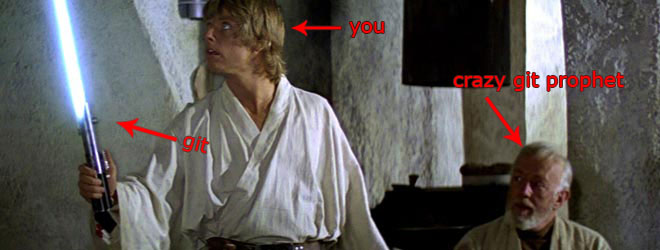
I was raised in an SVN household and later converted to Microsoft’s TFS. It was a conversion of convenience and I never developed the zeal of a true believer. I would listen to the followers of other systems proselytize their way, but never gave their way more than a cursory investigation.
Then these git guys started showing up.
They were different. They were weird. They didn’t want to talk about how git was better than SVN or TFS. They just wanted to talk about how much they loved git, and how much git loved them.
Intrigued by these crazy git prophets, I decided to embark on some source control tourism. I was going to try them all out. I was going to go back to the source control of my childhood (SVN), join these git guys for a while, finally get around to dropping by the Mercurial temple, and even go check out those Bazaar and Perforce sects I had heard about.
Two years later, I emerged a distributed source control convert who worships in the house of git.
Why I became a distributed source control convert
I am git disciple, but my true conversion was to the way of distributed source control systems. Mercurial, the other popular distributed option, is also a fantastic tool. Both of these tools essentially worship the same gods, but differ on how certain tenets of the faith should be followed. I am going to talk about git, but most of the points apply to Mercurial as well.
1. Git asks for little
Git doesn’t ask you to setup any special places of worship. You can practice git alone in your home, with a group, or in the beautiful GitHub cathedral. Git doesn’t care.
TFS and Perforce – on the opposite side of the spectrum – have very exacting requirements for their places of worship, which are extremely expensive and tedious to construct. SVN is certainly better then TFS or Perforce in this respect, but the setup and maintenance of a separate place of worship seems needlessly complex after git.
2. Git forgives much
Git is very forgiving. It is pretty hard to irreversibly screw something up in git. Even if you are tempted to try something insane (like rewriting history) backing up git is as simple as copying your .git
directory elsewhere.
This contrasts starkly with its centralized predecessors where backing up and restoring repositories is not trivial and mistakes are not easily forgiven. I am very wary of trying potentially destructive operations in these systems and as a result tend not to explore them as readily as I do git.
This forgiving nature removed any dread or trepidation I felt exploring the system and made git a lot of fun to learn.
3. Git sees all
IDEs like Visual Studio and Xcode have the disconcerting habit of asking if I want to save changes even if I am pretty sure I didn’t make any changes. Git restored my sense of sanity on these occasions. A simple status
lets me know which files have changes and a quick diff
shows exactly what changed.
The centralized brotherhood requires me to inform them when I want to check out a specific file and appear incredulous when I try to get them to understand that I’m not sure what changed.
IDE plugins for the centralized systems automate this notification, but should you edit a file outside the IDE and fail to inform central control, your change will not get picked up. As a result there is always an unsettling feeling in the back of your mind that there may be an orphaned change floating around out there.
Git’s omniscience gives you a warm fuzzy feeling that all is well and all changes are accounted for.
4. Git believes in many paths
Branching. The concept is supported by the centralized systems, but implemented as though branching is a serious and weighty decision that requires pre-branching counseling and a decision that should not be taken lightly. As a result, branching in these systems tends to be reserved for major changes.
Git treats branching like breathing. After you use git for a while branching will become an automatic reflex. Branching is so easy with these tools that it changed the way I actually write code.
Summary
Git is a wonderfully simple, flexible, and forgiving tool that just works. At first, to be honest, it’s a bit weird. Centralized systems are a lot easier to grok and the git merge model looks like a mess from the outside. But then you realize: development in the real world is a mess, and git works with that instead of against it.
The caveat of a novice
I can’t claim more than a novice’s understanding of all of the source control systems I tried on my trip. There might be some magical level of proficiency you can reach with the tool where the experience is transcended into bliss (I keep hearing that’s what finally mastering emacs is like). If it exists for TFS, SVN, or Perforce, I never reached it.